Web Development Best Practices for 2024
March 05, 2024
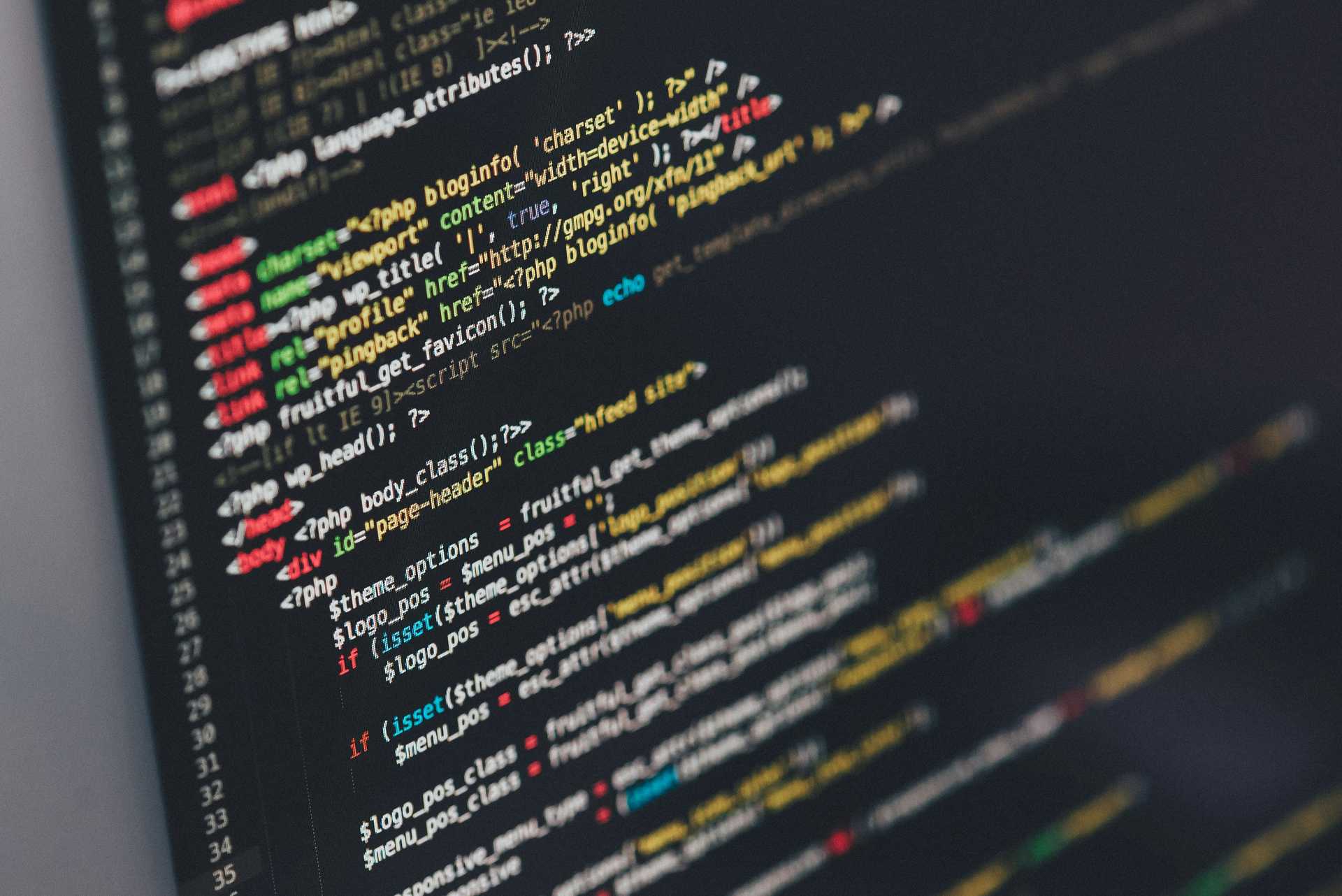
# Web Development Best Practices for 2024
As web development continues to evolve, staying up-to-date with best practices is crucial. Here's a comprehensive guide to the most important practices for 2024.
## 1. Performance Optimization
### Minimize Bundle Size
- Use code splitting
- Implement lazy loading
- Remove unused dependencies
```javascript
// Bad practice
import { everything } from 'huge-library'
// Good practice
import { onlyWhatINeed } from 'huge-library'
```
### Image Optimization
- Use modern formats (WebP, AVIF)
- Implement responsive images
- Lazy load images below the fold
```html
<img
src="image.webp"
srcset="image-300.webp 300w, image-600.webp 600w"
sizes="(max-width: 600px) 300px, 600px"
loading="lazy"
alt="Descriptive alt text"
/>
```
## 2. Accessibility (a11y)
### Semantic HTML
Use proper HTML elements for better accessibility:
```html
<!-- Bad practice -->
<div class="button" onclick="submit()">Submit</div>
<!-- Good practice -->
<button type="submit">Submit</button>
```
### ARIA Labels
Add ARIA labels when necessary:
```html
<button aria-label="Close modal">×</button>
```
## 3. Security Best Practices
### Input Validation
Always validate and sanitize user input:
```javascript
// Bad practice
const userInput = req.body.input;
db.query(`SELECT * FROM users WHERE id = ${userInput}`);
// Good practice
const userInput = sanitize(req.body.input);
db.query('SELECT * FROM users WHERE id = ?', [userInput]);
```
### Content Security Policy
Implement a strong Content Security Policy:
```html
<meta http-equiv="Content-Security-Policy"
content="default-src 'self';
img-src 'self' https://trusted-cdn.com;
script-src 'self';">
```
## 4. Modern CSS Practices
### Use CSS Custom Properties
```css
:root {
--primary-color: #007bff;
--secondary-color: #6c757d;
}
.button {
background-color: var(--primary-color);
}
```
### Implement CSS Grid and Flexbox
```css
.grid-layout {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 1rem;
}
```
## 5. Testing
### Unit Testing
Write comprehensive unit tests:
```javascript
describe('calculateTotal', () => {
it('should correctly calculate total with tax', () => {
expect(calculateTotal(100, 0.1)).toBe(110);
});
});
```
### End-to-End Testing
Implement E2E tests for critical user flows:
```javascript
describe('Login Flow', () => {
it('should successfully log in with valid credentials', () => {
cy.visit('/login');
cy.get('[data-testid="email"]').type('user@example.com');
cy.get('[data-testid="password"]').type('password123');
cy.get('[data-testid="submit"]').click();
cy.url().should('include', '/dashboard');
});
});
```
## Conclusion
Following these best practices will help you build better, more maintainable web applications. Remember to:
- Prioritize performance
- Make accessibility a core focus
- Implement proper security measures
- Use modern CSS features
- Write comprehensive tests
Stay tuned for more web development tips and best practices!